Syntax
public static double ChandelierStop(this Position p, Bars bars, int bar, int period, double coefficient )
public double ChandelierStop(Bars bars, int bar, Position p, int period, double coefficient )
Parameter Description
p | Position to apply Chandelier stop to |
period | TR period for Chandelier stop calculation |
coefficient | ATR factor for Chandelier stop calculation |
bars | Bars object |
bar | Bar number |
Description
The Chandelier Stop is a trailing stop that is based on the volatility of the market. It has been successfully used and recommended by a number of traders, including Chuck LeBeau, for trend-following systems.
This study implements following definition:
"The Chandelier Exit hangs a trailing stop from the highest high of the trade ... The distance from the high point to the trailing stop is measured in units of Average True Range".
Example
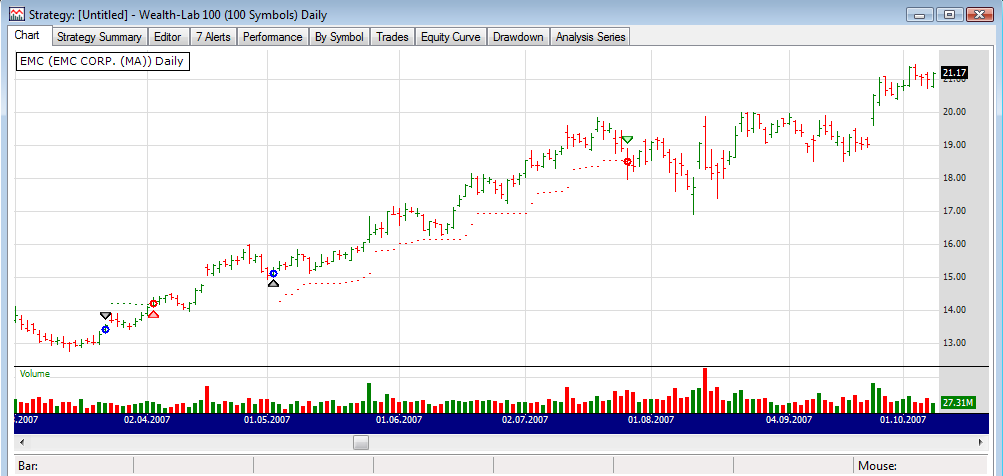 Chandelier Stop demonstration |
Below is a sample Strategy that uses the procedure.
Example using C# extension methods:
using System;
using System.Collections.Generic;
using System.Text;
using System.Drawing;
using WealthLab;
using WealthLab.Indicators;
namespace WealthLab.Strategies
{
public class ChandelierDemo : WealthScript
{
protected override void Execute()
{
// Variable that will hold Chandelier stop value
double chandelierStop = 0.0;
PlotStops(); // Ensure Chandelier stop visibility
for(int bar = Bars.FirstActualBar + 10; bar < Bars.Count; bar++)
{
if (IsLastPositionActive)
{
// Let's work directly with the list of active positions, introduced in WL5
for( int p = ActivePositions.Count - 1; p > -1 ; p )
{
Position pos = ActivePositionsp;
// Request Chandelier stop value using Period: 14, Coefficient: 3
chandelierStop = pos.ChandelierStop( Bars, bar, 14, 3 );
if( pos.PositionType == PositionType.Long )
SellAtTrailingStop( bar + 1, pos, chandelierStop, "Chandelier LX");
else
if( pos.PositionType == PositionType.Short )
CoverAtTrailingStop( bar + 1, pos, chandelierStop, "Chandelier SX");
}
}
else
{
if( DIPlus.Series( Bars, 10 )bar > 1.5 * DIMinus.Series( Bars, 10 )bar )
if( Lowbar <= KeltnerLower.Series( Bars, 10, 10)bar )
BuyAtMarket( bar + 1 );
if( DIMinus.Series( Bars, 10 )bar > 1.5 * DIPlus.Series( Bars, 10 )bar )
if( Highbar >= KeltnerUpper.Series( Bars, 10, 10)bar )
ShortAtMarket( bar + 1 );
}
}
}
}
}
Legacy syntax example:Make sure to complete the following checklist when implementing Chandelier stops in your own Strategy:
- Permit the use of Community.Components - the namespace that holds the ChandelierStop function:
- using Community.Components;
- Create an instance of the class that returns Chandelier stop value, passing an instance of the WealthScript class
- SeriesHelper obj = new SeriesHelper( this );
- Create a variable that will hold your Chandelier stop
- double chandelierStop = 0.0;
- In Position loop, call the ChandelierStop function to obtain the stop value
- chandelierStop = obj.ChandelierStop( Bars, bar, pos, 14, 3 );
- Outside of the main loop, call PlotStops to visualize your stop order levels
using System;
using System.Collections.Generic;
using System.Text;
using System.Drawing;
using WealthLab;
using WealthLab.Indicators;
using Community.Components; // includes Chandelier Stop
/*** Requires installation of Community.Components Extension from www.wealth-lab.com > Extensions ***/
namespace WealthLab.Strategies
{
public class ChandelierDemo : WealthScript
{
protected override void Execute()
{
// Create an instance of the class that returns Chandelier stop value
SeriesHelper obj = new SeriesHelper( this );
// Variable that will hold Chandelier stop value
double chandelierStop = 0.0;
PlotStops(); // Ensure Chandelier stop visibility
for(int bar = Bars.FirstActualBar + 10; bar < Bars.Count; bar++)
{
if (IsLastPositionActive)
{
// Let's work directly with the list of active positions, introduced in WL5
for( int p = ActivePositions.Count - 1; p > -1 ; p )
{
Position pos = ActivePositionsp;
// Request Chandelier stop value using Period: 14, Coefficient: 3
chandelierStop = obj.ChandelierStop( Bars, bar, pos, 14, 3 );
if( pos.PositionType == PositionType.Long )
SellAtTrailingStop( bar + 1, pos, chandelierStop, "Chandelier LX");
else
if( pos.PositionType == PositionType.Short )
CoverAtTrailingStop( bar + 1, pos, chandelierStop, "Chandelier SX");
}
}
else
{
if( DIPlus.Series( Bars, 10 )bar > 1.5 * DIMinus.Series( Bars, 10 )bar )
if( Lowbar <= KeltnerLower.Series( Bars, 10, 10)bar )
BuyAtMarket( bar + 1 );
if( DIMinus.Series( Bars, 10 )bar > 1.5 * DIPlus.Series( Bars, 10 )bar )
if( Highbar >= KeltnerUpper.Series( Bars, 10, 10)bar )
ShortAtMarket( bar + 1 );
}
}
}
}
}