Syntax
Community Indicators:
public SwingHiLo( Bars bars, int LeftBars, double LeftReversalAmount, int RightBars, double RightReversalAmount, double EqualPriceThreshold, bool PercentMode, bool SetLeftSwings, bool SetOuterSwings, bool SetSteppedSeries, string description )
public SwingHiLo( Bars bars, int LeftBars, int RightBars, double EqualPriceThreshold, bool SetLeftSwings, bool SetOuterSwings, bool SetSteppedSeries, string description )
public static SwingHiLo( Bars bars, int LeftBars, double LeftReversalAmount, int RightBars, double RightReversalAmount, double EqualPriceThreshold, bool PercentMode, bool SetLeftSwings, bool SetOuterSwings, bool SetSteppedSeries)
public SwingHi( DataSeries ds, int LeftBars, double LeftReversalAmount, int RightBars, double RightReversalAmount, double EqualPriceThreshold, bool PercentMode, bool SetLeftSwings, bool SetOuterSwings, bool SetSteppedSeries, string description )
public SwingHi( DataSeries ds, int LeftBars, int RightBars, double EqualPriceThreshold, bool SetLeftSwings, bool SetOuterSwings, bool SetSteppedSeries, string description )
public static SwingHi( DataSeries ds, int LeftBars, double LeftReversalAmount, int RightBars, double RightReversalAmount, double EqualPriceThreshold, bool PercentMode, bool SetLeftSwings, bool SetOuterSwings, bool SetSteppedSeries )
public SwingLo( DataSeries ds, int LeftBars, double LeftReversalAmount, int RightBars, double RightReversalAmount, double EqualPriceThreshold, bool PercentMode, bool SetLeftSwings, bool SetOuterSwings, bool SetSteppedSeries, string description )
public SwingLo( DataSeries ds, int LeftBars, int RightBars, double EqualPriceThreshold, bool SetLeftSwings, bool SetOuterSwings, bool SetSteppedSeries, string description )
public static SwingLo( DataSeries ds, int LeftBars, double LeftReversalAmount, int RightBars, double RightReversalAmount, double EqualPriceThreshold, bool PercentMode, bool SetLeftSwings, bool SetOuterSwings, bool SetSteppedSeries )
Community Components:
public static bool IsSwingHi(int bar, DataSeries ds, int LeftBars, double LeftReversalAmount, int RightBars, double RightReversalAmount, int farback, int occur, double EqualPriceThreshold, bool PercentMode, bool SetLeftSwings, out int swHighBar)
public static bool IsSwingHi(int bar, DataSeries ds, int LeftBars, int RightBars, int farback, int occur, double EqualPriceThreshold, bool SetLeftSwings, out int swHighBar)
public static bool IsSwingLo(int bar, DataSeries ds, int LeftBars, double LeftReversalAmount, int RightBars, double RightReversalAmount, int farback, int occur, double EqualPriceThreshold, bool PercentMode, bool SetLeftSwings, out int swLowBar)
public static bool IsSwingLo(int bar, DataSeries ds, int LeftBars, int RightBars, int farback, int occur, double EqualPriceThreshold, bool SetLeftSwings, out int swLowBar)
Parameter Description
Community Indicators:bars | source Bars inc High & Low series |
ds | source DataSeries |
LeftBars | number of bars left of the swing high or low bar for true |
RightBars | number of bars right of the swing high or low bar for true |
LeftReversalAmount | price or percent value left of the swing high or low bar for true |
RightReversalAmount | price or percent value right of the swing high or low bar for true |
EqualPriceThreshold | a value below which the source prices will be deemed equal |
PercentMode | true for reversal amounts in percent, false for price |
SetLeftSwings | to select left bar in cases of equal price swing bars |
SetOuterSwings | to select bar outside of consequtive swings in cases of equal price bars |
SetSteppedSeries | plot as stepped instead of incremental series |
Extra parameter descriptions for
Community Components:
bar | from which to search for required swing occurrence |
farback | how many past bars to search |
occur | which of the 1st, 2nd, 3rd and so on, most recent swings to return |
swHighBar | assign an existing int variable with the bar number of 'occur'. The C# keyword ‘out’ must be included in the parameter list. |
swLowBar | assign an existing int variable with the bar number of 'occur'. The C# keyword ‘out’ must be included in the parameter list. |
Description
A stable of indicators and methods for defining swings by Jon Macmichael.
The indicators do not have a value method. Instead use the Community.Components IsSwingLo() & IsSwingHi() methods.
Swings can be defined assymetrically by price or percent as well as bars, and plotted in a stepped or incremental style. There are further controls for cases of multi bar highs & lows. The associated methods provide further controls for the the number of bars to look back for a swing, and which occurrence.
When both bar quota and price are used the early(left) history will require a match from both parameters, whereas the recent(right) history will be matched when either parameter is fulfilled.
To drop price-percent filtering set their parameter(s) to zero, or use an overload. Alternatively, to drop the bars quota set the left side parameter to zero, but on the right side set its parameter to a minimum of one.
For cases of multi-bar swings the default bar returned is the right side. This can be overridden with the SetLeftSwings parameter. Alternatively the parameter
SetOutSideSwings will force the outside bar of multi-bar swings to be returned (in cases of consecutively higher(lower) swings.
The SetSteppedSeries parameter will force only the last swings values to be plotted box style. The default plot increments the series values between swings.
These series display 'peaked' values from the (right side) swing bar until the bar since that is true to the RightBars or RightReversalAmount value. To overcome this, the returned series could be used inconjunction with associated methods IsSwingHi() and IsSwingLo(). Alternative a series could be built completely using these methods.
The SwingHiLo series has an unavoidable logic bug in cases of a bar matching both swing high and low parameters.
Extra Description for Community.Components
If the bool methods IsSwingHi() and IsSwingLo() do not return a match(false), then -1 is returned in their ‘out’ prameters.
The minimum value for ‘farback’ should be RightBars + 1.
Example
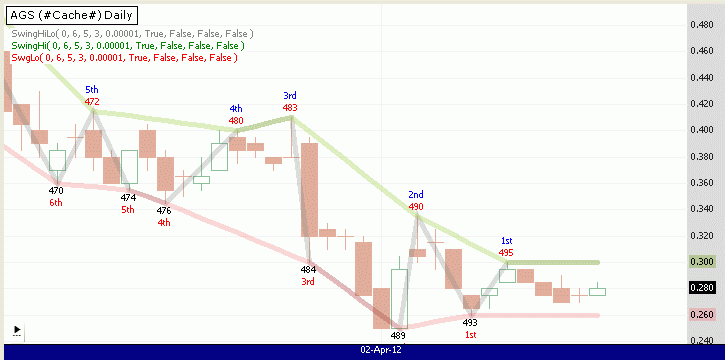 |
using System;
using System.Collections.Generic;
using System.Text;
using System.Drawing;
using WealthLab;
using WealthLab.Indicators;
using Community.Indicators;
using Community.Components;
/*** Requires installation of Community.Components Extension from www.wealth-lab.com > Extensions ***/
namespace WealthLab.Strategies {
using sw = IsSwing;
public class MyStrategy : WealthScript {
#region - parameter sliders & public methods -
private StrategyParameter sliLeft;
private StrategyParameter sliLeftPrice;
private StrategyParameter sliRight;
private StrategyParameter sliRightPrice;
private StrategyParameter sliPercent;
private StrategyParameter sliOccur;
private StrategyParameter sliUseLeft;
private StrategyParameter sliUseOutside;
private StrategyParameter sliBoxedSeries;
public MyStrategy()
{
sliLeft = CreateParameter("left bars", 13, 0, 30, 1);
sliLeftPrice = CreateParameter("left price", 3.5, 0, 100, 0.001);
sliRight = CreateParameter("right bars", 5, 1, 30, 1);
sliRightPrice = CreateParameter("right price", 1.5, 0, 100, 0.001);
sliPercent = CreateParameter("use percent chg", 1, 0, 1, 1);
sliOccur = CreateParameter("occurence", 5, 1, 25, 1);
sliUseLeft = CreateParameter("use left swing", 0, 0, 1, 1);
sliUseOutside = CreateParameter("use ouside swings", 0, 0, 1, 1);
sliBoxedSeries = CreateParameter("use boxed series", 0, 0, 1, 1);
}
string suffx(int ctr) {
int th = ctr == 11 || ctr == 12 || ctr == 13 ? -1 : ctr % 10;
string ret = "";
switch (th)
{
case 1 :
ret = "st";
break;
case 2 :
ret = "nd";
break;
case 3 :
ret = "rd";
break;
default:
ret = "th";
break;
}
return ret;
}
#endregion
protected override void Execute() {
#region - variables & initial settings -
PadBars(4);
HideVolume();
//SetBarColors( Color.Green, Color.Red);
int Lbars = sliLeft.ValueInt;
double Lprice = sliLeftPrice.Value;
int Rbars = sliRight.ValueInt;
double Rprice = sliRightPrice.Value;
bool UsePercent = sliPercent.ValueInt == 0 ? false : true;
bool UseLeft = sliUseLeft.ValueInt == 0 ? false : true;
bool UseOutsideSwings = sliUseOutside.ValueInt == 0 ? false : true;
bool UseBoxed = sliBoxedSeries.ValueInt == 0 ? false : true;
int swgLoBar;
int swgHiBar;
int lastLoBar = 999999;
int lastHiBar = 999999;
int _occur = sliOccur.ValueInt;
string _params = String.Format("{0}, {1}, {2}, {3}, {4}, {5}, {6}, {7}, {8}",
Lbars, Lprice, Rbars, Rprice, "0.00001", UsePercent, UseLeft, UseOutsideSwings, UseBoxed);
#endregion
DataSeries SwgHiLo = SwingHiLo.Series(Bars, Lbars, Lprice, Rbars, Rprice, 0.00001, UsePercent, UseLeft, UseOutsideSwings, UseBoxed);
DataSeries SwgHi = SwingHi.Series(High, Lbars, Lprice, Rbars, Rprice, 0.00001, UsePercent, UseLeft, UseOutsideSwings, UseBoxed);
DataSeries SwgLo = SwingLo.Series(Low, Lbars, Lprice, Rbars, Rprice, 0.00001, UsePercent, UseLeft, UseOutsideSwings, UseBoxed);
SwgHiLo.Description = "SwingHiLo( " + _params + " )";
SwgHi.Description = "SwingHi( " + _params + " )";
SwgLo.Description = "SwgLo( " + _params + " )";
PlotSeries(PricePane, SwgHiLo, Color.FromArgb(70, Color.Gray), WealthLab.LineStyle.Solid, 5);
PlotSeries(PricePane, SwgHi, Color.FromArgb(80, Color.YellowGreen), WealthLab.LineStyle.Solid, 5);
PlotSeries(PricePane, SwgLo, Color.FromArgb(40, Color.Red), WealthLab.LineStyle.Solid, 5);
DrawLabel(PricePane, SwgHiLo.Description, Color.Gray);
DrawLabel(PricePane, SwgHi.Description, Color.Green);
DrawLabel(PricePane, SwgLo.Description, Color.Red);
// 1st Occurence looping backward each bar
for(int ii = Bars.Count - 1; ii > Lbars + Rbars + 1 && ii > Bars.Count - 500; ii ) {
if( sw.IsSwingLo( ii, Low, Lbars, Lprice, Rbars, Rprice, Rbars + 1, 1, 0.00001, UsePercent, UseLeft, out swgLoBar) ) {
if( swgLoBar